-
Need for Programmers and Programming
-
Definition of Compiler
Tutors
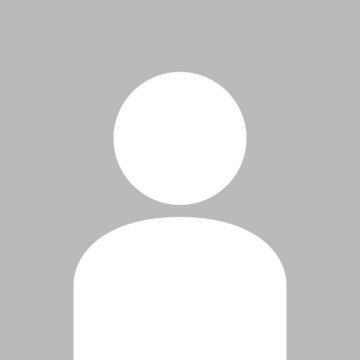
The Diploma
PROGRAM OVERVIEW
Description:
Advanced java is used for developing the web based application and enterprise application. It will take you through Java features, OOPS concept, threading, exception, package, collections, JDBC concept. These all are very basic concept and using this you can develop the simple stand-alone applications
Advance java comprises mainly of three things:
JSP: JSP stands for Java Server Pages. JSP is used to build up forms. It’s used as a view part of the MVC model. if somebody don’t know to wrote the code in JSP they can directly use JavaScript or HTML with CSS
SERVLET: servlet is the controller part of MVC model. This is used to read the content of data whatever is coming from the view part. Eg: reading form data . I someone doesn’t know how to write servlet code then they can go for frameworks like Spring MVC
JDBC: this is the model part of the MVC model. This is used for getting connected with database and performing database operations like storing and retrieving data. if someone doesn’t know how to write database code then they can go for a framework called Hibernate. Other frameworks include Spring, Apache Struts, Spark etc.
What will you Learn?
In Advanced java you will learn about
OOPS concept
collections
Generic programming
Sequential and associative data structures
Classic data structures
Sorting and searching
Exception handling
Database programming with JDBC
JDBC concept
Networking programming GUI development using Swing
An overview of Multithreading.
You will also explore Java Applets, web applications (Servlets), advanced input and output classes, more advanced strings, regular expressions, Java graphics, and using Eclipse.
Prerequisites:
We need to learn basic language on C, C++, Core JAVA
We need to learn Object Oriented Programming Concepts.
We have to know good Programming and Analytical Skills.
CURRICULUM
Introduction to Programming Languages
Programming language Types and Paradigms
-
Procedural Oriented Programming Paradigm
-
Object Oriented Programming Paradigm
-
Overview of OOPS
Evolution of Programming Languages
-
History and Influence of Programming Languages
-
Introduction to Evolution of Programming Languages
-
Low Level Programming Languages
-
Middle Level Programming Languages
High Level Programming Languages
-
ALGOL
-
BCPL and B Language
-
K & R and ANSI C
-
Fortran
-
Pascal and Cobol
-
C Language
-
C++
-
Java
History of Java
-
Java History
-
Why OAK?
-
Why OAK Renamed as Java?
-
Why Java Logo was Coffee Cup?
-
Java Version History
-
Is Java Object Oriented Programming Language?
Introduction to Java
-
About Java
-
Why we Use Java?
-
Where we use Java in Real Wold?
-
The Java Programming Language
-
Software Code Compilation and Execution
-
Characteristics and Features of Java
Overview of Java
-
Overview of Software Development Process
-
Java Design Goal
-
JRE, JDK, and JVM
-
Why Java is Slow?
-
Why Java Divided into Advanced and Core Java?
-
Java Suite
Environment and Installation of Java
-
Environment of Java
-
Check if Java is Already Installed?
-
Downloading JDK
-
Installing JDK and JRE
-
Setting Path and Verifying JDK Installation
-
Compiling and Running a Java Program
Developing a Java Program
-
Developing a Java program
-
Viewing Class Files
-
Javac Compilation Process
Introduction to Advanced Java Programming
-
Introduction to Advanced Java
-
Real World Java Applications
Web Applications in Java
-
Introduction to Web Applications
-
Web Server and Web Client
-
HTML and HTTP
-
Why we Need Servlet and JSP
Recap of Core Java : Module - 1
-
File Structure
-
Main Method in Java
-
Editing, Compiling and Executing a Java Program
-
Classes and Objects in Java
-
Constructors
-
Import
Recap of Core Java : Module - 2
-
Inheritance
-
Polymorphism, Method Overloading and Overriding
-
Primitive Data Types in Java
-
Variables, Operators and Arrays in Java
Recap of Core Java : Module - 3
-
Access Modifier
-
Static Modifier
-
Final Modifier
-
Abstract Modifier
Recap of Core Java : Module - 4
-
Interfaces
-
Flow Control
-
Constructors
-
Exception Handling
Database Applications
-
Introduction to Database Applications
-
About SQL (Structured Query Language)
-
SQL Commands
Installation Procedure of ECLIPSE IDE
-
Introduction
-
Downloading ECLIPSE IDE
-
Creating an ECLIPSE Project
-
First Program in ECLIPSE IDE
Installation Procedure of ORACLE XE
-
Installation Procedure of ORACLE XE
Installation Procedure of Java
-
Installation Procedure of Java
JDBC (Java Data Base Connectivity)
-
Introduction to JDBC
-
Introduction to JDBC Architecture
-
Introduction to JDBC Drivers
-
Classes and Interfaces
-
Using JDBC
-
JDBC Architecture Lecture - 1
-
JDBC Architecture Lecture - 2
-
JDBC Architecture Lecture - 3
-
Interfaces in JDBC API
-
JDBC Drivers
-
JDBC - ODBC Bridge Drivers
-
Native - API Driver
-
Network Protocal Driver
-
Thin Driver
-
JDBC Driver Manager
Arrays
-
Arrays Lecture -1
-
Arrays Lecture -2
-
Arrays Lecture -3
-
Arrays Lecture -4
Clone Method In Java
-
Clone Method in Java