-
Need for Programmers and Programming
-
Definition of Compiler
Tutors
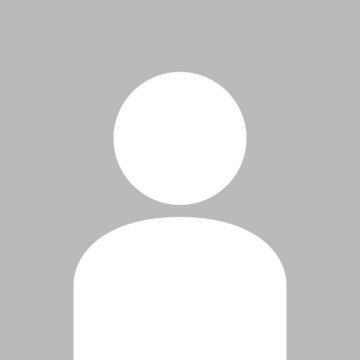
The Diploma
PROGRAM OVERVIEW
Description:
Java was designed to have the look and feel of the C++ language, but it is simpler to use than C++ and enforces an object-oriented programming model. Java can be used to create complete applications that may run on a single computer or be distributed among servers and clients in a network. It can also be used to build a small application module or applet for use as part of a webpage.
Java platforms:
There are three key platforms upon which programmers develop Java applications:
Java SE:
Simple, stand-alone applications are developed using Java Standard Edition. Formerly known as J2SE, Java SE provides all of the APIs needed to develop traditional desktop applications.
Java EE:
The Java Enterprise Edition, formerly known as J2EE, provides the ability to create server-side components that can respond to a web-based request-response cycle. This arrangement allows the creation of Java programs that can interact with internet-based clients, including web browsers, CORBA-based clients and even REST- and SOAP-based web services.
Java ME:
Java also provides a lightweight platform for mobile development known as Java Micro Edition, formerly known as J2ME. Java ME has proved a very popular platform for embedded device development, but it struggled to gain traction in the smartphone development arena. In terms of smartphone development, Android has become the mobile development platform of choice.
What will you Learn?
To be a great programmer, you must assimilate endless knowledge - be it conceptual or theoretical. Java is a complex language and needs hard hitting mindsets.
You must know core concepts of Java well, which includes:
Variables
Fields
Constructors
Classes + Objects
Packages
Methods
Interfaces
Operations
Arrays
Then you should move on to advanced concepts like:
OOPs concept
Date and time handling API
Internal structure of data structure
Exception handling
Multithreading
Inner classes
File handling (I/O)
Collection framework
String handling
Generics Lambdas and streams, the newer concept in JAVA
Prerequisites:
We need to learn basic language on C, C++
We need to learn Object Oriented Programming Concepts.
We have to know good Programming and Analytical Skills.
We have to know about Loops Concepts.
CURRICULUM
Introduction to Programming Languages
Programming Language Types and Paradigms
-
Procedural Oriented Paradigm
-
Object Oriented Paradigm
-
Overview of OOPS
Evolution of Programming Languages
-
History and Influence of Programming Languages
-
Evolution of Programming Languages
-
Low Level Programming Languages
-
Middle Level Programming Languages
High Level Programming Languages
-
ALGOL
-
BCPL and B Language
-
K & R and ANSI C
-
FORTRAN
-
PASCAL and COBOL
-
C Language
-
C++
-
JAVA
History of Java
-
Java History
-
Why OAK ?
-
Why OAK Renamed as Java ?
-
Why Java Logo is Coffee Cup ?
-
Versions of Java
-
Is Java Object Oriented Programming Language ?
Introduction to Java
-
About Java
-
Why we Use Java?
-
Java in Real World
-
Working of Java Program
Overview of Java
-
Overview of Software Development Process
-
Java Platform
-
Java Design Goal
-
JRE, JDK, and JVM
-
Software Code Compilation and Execution Process
-
Why Java is Slow?
-
Why Java Divided into Core and Advanced Java
-
Java Suite
-
Characteristics and Features of Java
Environment of Java
-
Java Environment
-
Compiling and Running a Java Program Before Java JDK Installation
Installation Procedure
-
Check if Java is Already Installed?
-
Downloading JDK
-
Installing JDK and JRE
-
Setting Path and Verifying JDK Installation
Developing a Java Program
-
Developing a Java Program
-
Viewing Class Files
-
Javac Compilation Process
Java Language Fundamentals
-
Standard JDK Tools and Utilities
-
Set the Memory Available to the JVM
-
Java Source File Structure
Basic Language Elements
-
Lexical Elements of Java
-
Fundamental Building Blocks
-
Class, Objects and Methods in Java Arrays
-
Interfaces and Arrays in Java
Java Tokens
-
Introduction to Java Tokens
-
Identifiers
-
Keywords
-
Separators
-
Comments and Whitespaces
-
Operators
Data Types
-
Primitive Data Types
-
Overflow
-
Wrapper Object
Java Programs on Data Types
-
Program on Boolean and Byte
-
Program on Primitive Data Types
-
Program on Static Initialization for Data Types
-
Program on Dynamic Initialization for Data Types
-
Program on Limits of Primitive Data Types
-
Program on Lifetime of a Variable
-
Program on Default Initial Values
Type Casting
-
Introduction to Type Casting
-
Widening Type Casting
-
Narrowing Type Casting
Variable Scope
-
Introduction to Variable Scope
-
Rules of Variable Scope
-
Class Level and Method Level Variables
Unary Operators
-
Introduction to Operators
-
Increment and Decrement Operators
-
Prefix and Postfix Operators
Java Programs on Unary Operators
-
Program on Post Increment Demo
-
Program on Post Decrement Demo
-
Program by using ++ And -- with Floating Point Variables
Binary Operators
-
Arithmetic Operators
-
Assignment Operators
-
Relational Operators
-
Logical Operators
Java Programs on Binary Operators
-
Program on Arithmetic Operators
-
Program on Assignment Operators
-
Program on Relational Operators
-
Program on Logical OR Operator
-
Program on Logical AND Operator
Ternary Operator
-
Introduction to Ternary Operator
Bitwise Operators
-
Introduction to Bitwise Operator
-
Bitwise AND, XOR, Shift, Complement
-
&& Versus & Operator
Java Programs on Bitwise Operators
-
Program on Bitwise Logical Operators
-
Program on Bitwise Assignment and Left Shifting
Special Operators
-
Modulus Operator
-
Short Circuiting Behavior
-
Program on Short Circuiting Behavior
-
Comma Operator
-
The op= Operators
-
Miscellaneous (Misc) Operator
Instanceof Operator
-
Introduction to Instanceof Operator
-
Usage of Instanceof Operator
-
Class Hierarchy
Java Programs on Operators
-
Program on All Operators : Lecture - 01
-
Program on All Operators : Lecture - 02
-
Program on All Operators : Lecture - 03
-
Program on All Operators : Lecture - 04
Constructors
-
Constructors in Java
Strings in Java
-
Lecture -1
-
Lecture -2
Arrays in Java
-
Arrays Lecture -1
-
Arrays Lecture -2
-
Arrays Lecture -3
-
Arrays Lecture -4
Clone Method in Java
-
Clone Method in Java