-
Programming Languages Paradigm
-
Imperative Paradigm
-
Declarative Paradigm
-
Structured Programming
-
Procedural Programming
-
Object Oriented Programming
-
Functional Programming
Tutors
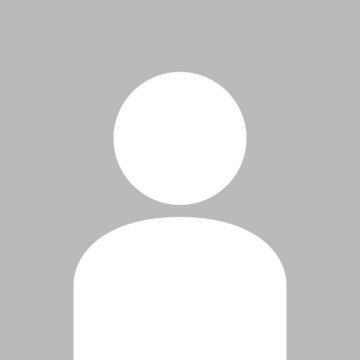
The Diploma
PROGRAM OVERVIEW
Description:
C# is a general object-oriented programming (OOP) language for networking and Web development. C# is specified as a common language infrastructure (CLI) language.
In January 1999, Dutch software engineer Anders Hejlsberg formed a team to develop C# as a complement to Microsoft’s NET framework. Initially, C# was developed as C-Like Object Oriented Language (Cool). The actual name was changed to avert potential trademark issues. In January 2000, NET was released as C#. Its NET framework promotes multiple Web technologies.
The term’s # character derives its name from the musical sharp key, which denotes a one semitone pitch increase. C# is pronounced "see sharp."
C# improved and updated many C and C++ features, including the following:
1. C# has a strict Boolean data variable type, such as bool, whereas C++ bool variable types may be returned as integers or pointers to avoid common programming errors.
2. C# automatically manages inaccessible object memory using a garbage collector, which eliminates developer concerns and memory leaks.
3. C# type is safer than C++ and has safe default conversions only (for example, integer widening), which are implemented during compile or run time.
No implicit conversions between Booleans, enumeration members and integers (other than 0) may be converted to an enumerated type. User-defined conversions must be specified as explicit or implicit, versus the C++ default implicit conversion operators and copy constructors.
What will you Learn?
1. Learning of C# language and .NET Framework
2. Fundamentals of C#
3. Build logic to programming in C#
4. Demonstration of C# Features
5. Step wise learning of C# language with building logic
6. Details of control statements with logic to write programs
7. Variety of programs to have great programming skill
8. Introduction of class and object
Prerequisites:
1. Basic understanding of Programming language.
2. Basic understanding of Programming environment.
3. Visual Studio IDE to write, compile and run the program.
CURRICULUM
Introduction to Programming Languages
History of Programming Language
-
History of Programming Language
Classification of Programming Languages
-
Low Level Programming Language
-
Middle Level Programming Language
-
High Level Programming Language
High level programming languages
-
FORTRAN
-
ALGOL
-
COBOL
-
BCPL
-
PASCAL
-
B Language
-
C Language
-
C++
-
Java
Introduction to C#
-
Why it is Named as C#
-
Is C# Platform Dependent or Independent
Features of C#
-
Introduction to Features of C#
-
Simple
-
Modern Programming Language
-
Object Oriented
-
Type Safe
-
Interoperability
-
Scalable and Updatable
-
Component Oriented
-
Structured Programming Language
-
Rich Library
Versions of C#
-
Versions of C#
Importance of C#
-
Advantages of C#
-
What will you gain if you learn C#?
History of C#
-
Beginning of Modern Age Programming
-
Creation of OOPS and C++
-
Internet and Java Emerge
-
Creation of C#
-
Evolution of C#
-
How C# Relates to the .NET Framework
-
.NET Framework and its Components
Your First Program
-
First Program using Command Line Compiler
-
First Program Using Visual Studio IDE
-
Handling Syntax Errors
-
Debugging
Basic Syntax
-
Semicolons
-
Keywords
-
Identifiers
Data Types
-
Introduction to Data Types
-
Different Data Types in C#
-
Value Data Type
-
Reference Data Type
-
Pointer Data Type
-
Why Data Types are Important
Literals & Variables
-
Literals
-
Variables
-
Initializing Variables
-
Scope of Variables
Operators
-
Introduction to Operators
-
Arithmetic Operator
-
Increment & Decrement Operator
-
Bitwise Operator
-
Ternary Operator
-
Spacing & Parenthesis
-
Operator Precedence
Conditional Statements
-
If Statement
-
If-else Statement
-
If-else if Statement
-
Nested if-else Statement
-
Switch Statement
-
Nested Switch Statement
Jump Statements
-
goto Statement
-
break Statement
-
continue Statement
-
return Statement
Iteration Statements
-
While Statement
-
Do-while Statement
-
For Statement
-
For each Statement
-
Some Variations on For Loop
Overview of OOPS
-
Introduction to OOPS
-
Class
-
Object
-
Abstraction
-
Encapsulation
-
Inheritance
-
Polymorphism
Classes
-
Introduction to Class
-
Rules for Declaring Class Name
-
Access Specifiers or Modifiers
-
Generalized program for Access Specifier or Modifier
-
Internal & Protected
-
Generalized program for a Class
Objects
-
Introduction to Object
-
Creating an Object
-
Releasing an Object
-
Declaring & Instantiating Object
-
Access to fields of an Object
Methods
-
Introduction to method
-
Method Deceleration
-
Return Type Method
-
Non - Return Type Method
Types of Methods
-
Pure Virtual Method
-
Virtual Method
-
Abstract Method
-
Partial Method
-
Extension Method
-
Instance Method
-
Static Method
Passing Parameter to a Method
-
Introduction to Passing Parameter to a Method
-
Value as a Parameter
-
Reference as a Parameter
-
Output as a Parameter
Method Overloading
-
Introduction to Method Overloading
-
By Changing the Number of Parameters
-
By Changing the Data Types of the Parameters
-
By Changing the Order of the Parameters
Constructors
-
Introduction to Constructor
-
Rules for Declaring a Constructor
Types of Constructor
-
Default Constructor
-
Parametarized Constructor
-
Copy Constructor
-
Private Constructor
-
Static Constructor
Destructors
-
Introduction to Destructor
-
Rules for Declaring a Destructor
-
Garbage Collector