-
Programming & Data Structures
-
Fibonacci Series
-
Introduction to Data Structures
Tutors
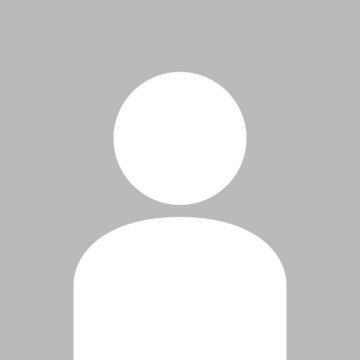
The Diploma
PROGRAM OVERVIEW
Description
A data structure is a particular way of organizing data in a computer so that it can be used effectively. The idea is to reduce the space and time complexities of different tasks. Use a data structure to store the values and groups of values in your applications. General data structure types include the array, the file, the record, the table, the tree, and so on. Any data structure is designed to organize data to suit a specific purpose so that it can be accessed and worked with appropriate ways.
What will you learn?
Basic concepts of Algorithm Specification, Data Abstraction, Perform time complexity and space complexity, Asymptotic Notation, introduction to Linear and Non-Linear data structures. Singly Linked Lists, concatenating singly-linked lists, circularly linked lists, Doubly Linked Lists, Representation of single, two array and linked representations, Stacks, Queues, Trees, Graphs, Searching and Sorting, etc.
Prerequisites
1. Introduction to any programming language mostly C.
2. Basic mathematics and algebra
3. Basic awareness about language syntax and semantics
4. Good problem-solving abilities.
CURRICULUM
Introduction to Programming & Data Structures
Memory Allocation
-
Heap Memory
-
Dynamic Memory
Arrays
-
Arrays
-
2D Arrays
-
3D Arrays
-
4D Arrays
-
Horner's Rule
-
Arrays - Q and A
Pointers
-
Pointers
-
Size of Pointer
-
Void Pointer
-
Function Pointer
Problem Solving on Programming
-
Problem Solving on Programming Lecture 1
-
Problem Solving on Programming Lecture 2
-
Problem Solving on Programming Lecture 3
-
Problem Solving on Programming Lecture 4
-
Problem Solving on Programming Lecture 5
Structures and Unions
-
Structure
-
Union
Linked List - Module 01
-
Linked List - Lecture 01
-
Linked List - Lecture 02
-
Examples of Linked List
-
Circular Linked List
-
Circular Doubly Linked List
Linked List - Module 02
-
Doubly Linked List - Lecture 01
-
Doubly Linked List - Lecture 02
-
List
-
Linked List - Level 01 Questions
-
Linked List - Level 02 Questions
Stacks - Module 01
-
Data Structures - Stack
Stacks - Module 02
-
Application of Stack
-
Infix to Postfix Conversion
-
Infix to Postfix Conversion Using Stack
-
Evalution of Postfix
Queues
-
Queues
-
Queues using Arrays
-
Circular Queue
-
Queue using Linked List
-
Queue using Stacks
-
Queue using 2 stacks
Tree
-
Tree
-
Heights vs Nodes
-
Complete N - Array Tree
-
Recursion and Tree Recursion - Lecture 01
-
Recursion and Tree Recursion - Lecture 02
Tree - Binary Tree
- This section has no content published in it.
Tree - Traversal Methods
- This section has no content published in it.
Tree - AVL Tree
- This section has no content published in it.
Heaps
- This section has no content published in it.
Sorting Techniques - Module 01
- This section has no content published in it.
Sorting Techniques - Module 02
- This section has no content published in it.
Problem solving on Stacks and Queues
- This section has no content published in it.
Problem Solving on Tree
- This section has no content published in it.
Problem Solving on Graphs
- This section has no content published in it.